Need for Speed: YARV v. Node
On my first day learning javascript, I kept hearing / reading how much ‘faster’ my javascript programs would run compared to the Ruby programs I was accustomed to. One of the main reasons for the increased speed is V8, Google’s open source high-performance JavaScript engine. I decided the best way to put my new found knowledge to the test was to have a drag race. I decided to use the first Project Euler problem as my drag strip, and node.js and YARV as my race cars.
The Problem
If we list all the natural numbers below 10 that are multiples of 3 or 5, we get 3, 5, 6 and 9. The sum of these multiples is 23. Find the sum of all the multiples of 3 or 5 below 1000.
Code
I wanted to make my Ruby code and Javascript code as similar as possible. Both solutions increment a count, test if it is divisible by 3 or 5, add the current count to a total if it is divisible and finally increment the count by 1. This process is repeated until the limit (in our case 1000) is reached.
Ruby
require 'benchmark' total = 0 execution_time = Benchmark.realtime{
x = 3 until x >= 1000 if x % 3 == 0 || x % 5 == 0 total += x end x += 1 end} puts "The sum is #{total}" puts "Execution Time: #{"%1.12f" % execution_time} seconds"
Javascript
var aTimer = process.hrtime(); var sum = 0; for (var x = 3 ; x < 1000 ; x++) { if (x % 3 === 0 || x % 5 === 0) { sum += x; } } console.log('The sum of them is: '+ sum); var aTimerDiff = process.hrtime(aTimer); console.info("Execution time: %dseconds", aTimerDiff[1]/1000000000);
First Race: Limit => 1000
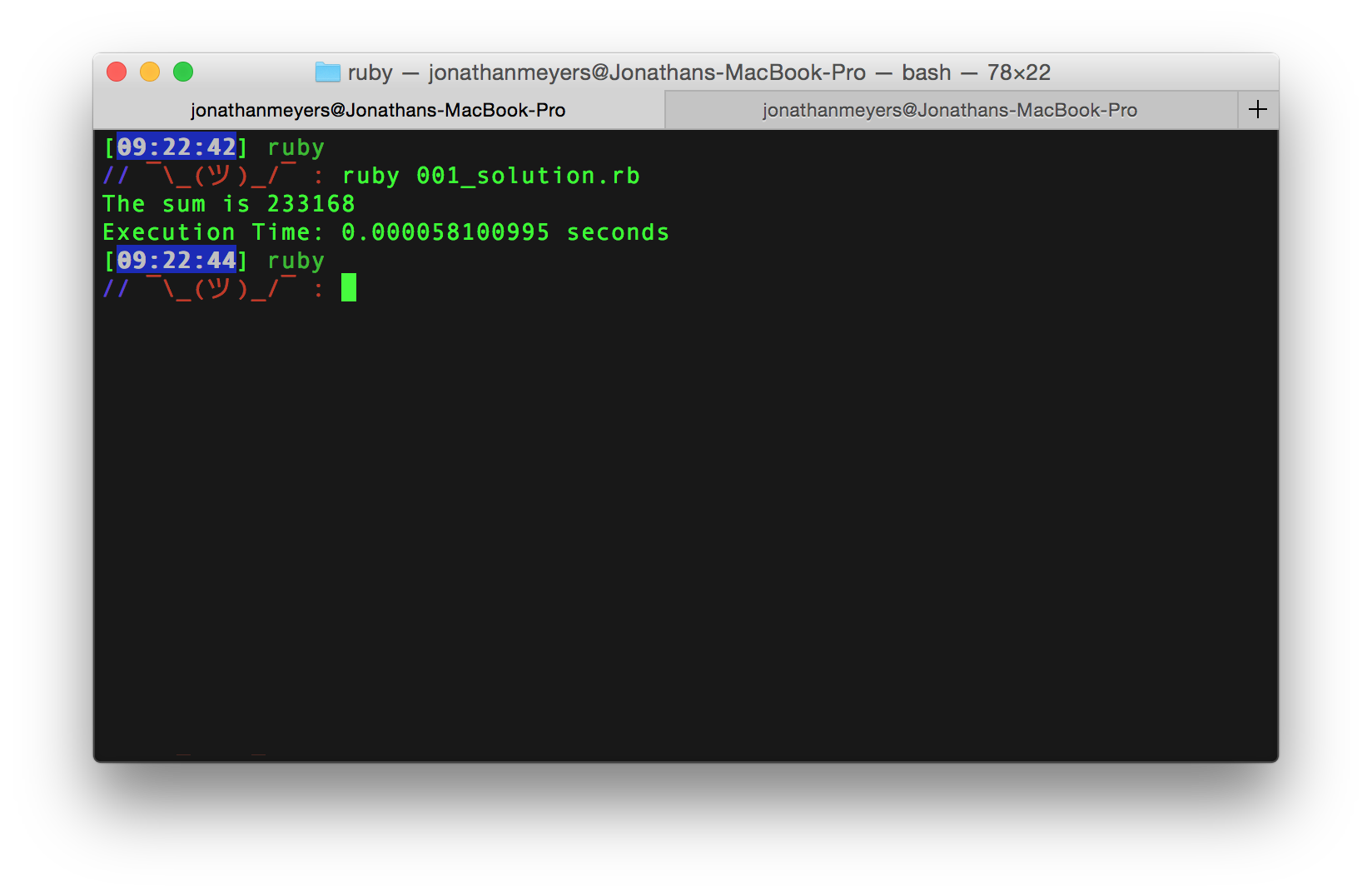
V.
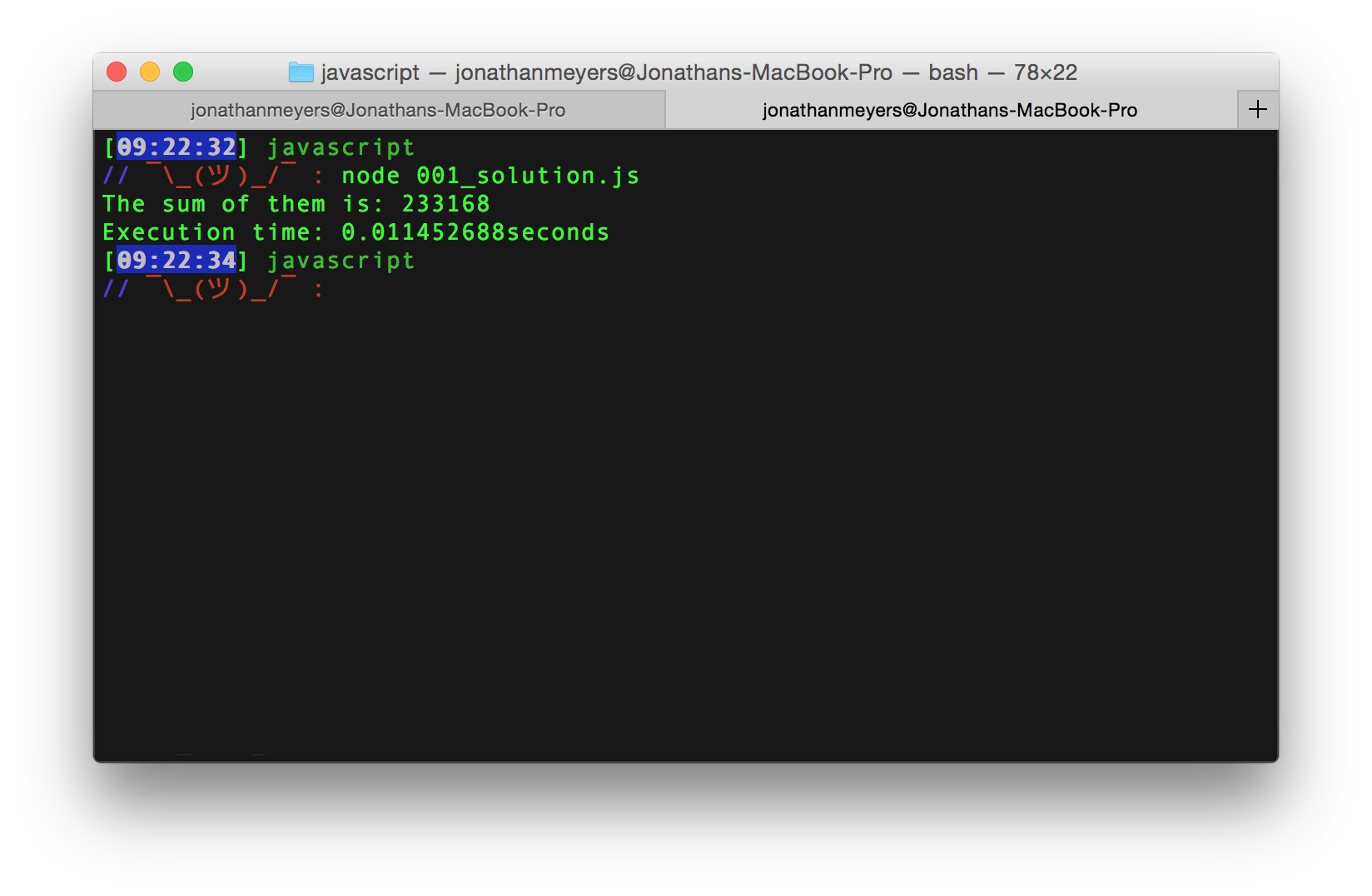
To my surprise, Ruby was faster…Much. Faster. Were all of the speed optimizations of Javascript an exageration? Had Ruby made Earth shattering speed improvements when it switched from MRI to YARV in Ruby 1.9? I needed another test, this time with a much larger calculation…
Second Race: Limit => 100000000
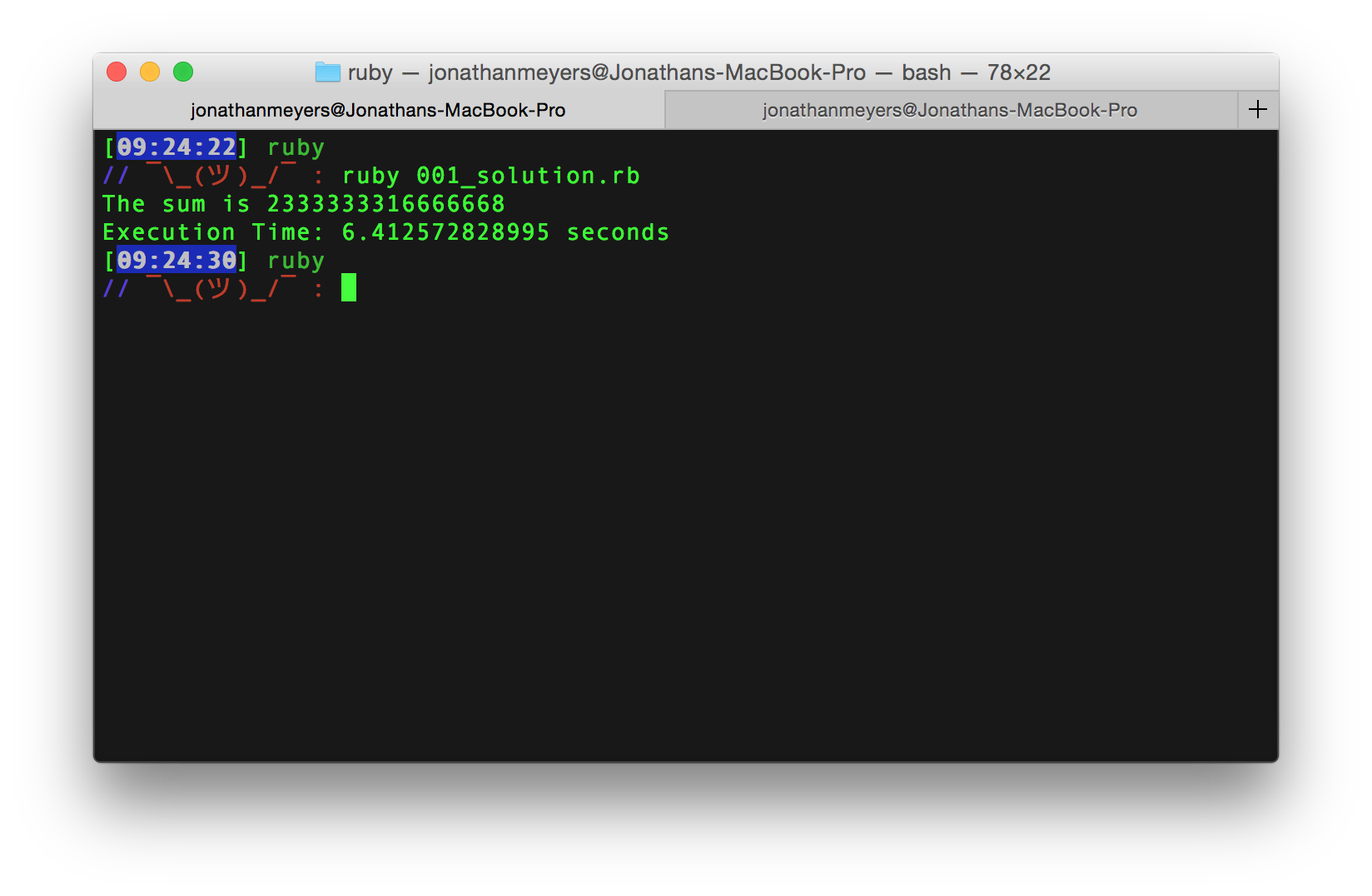
V.
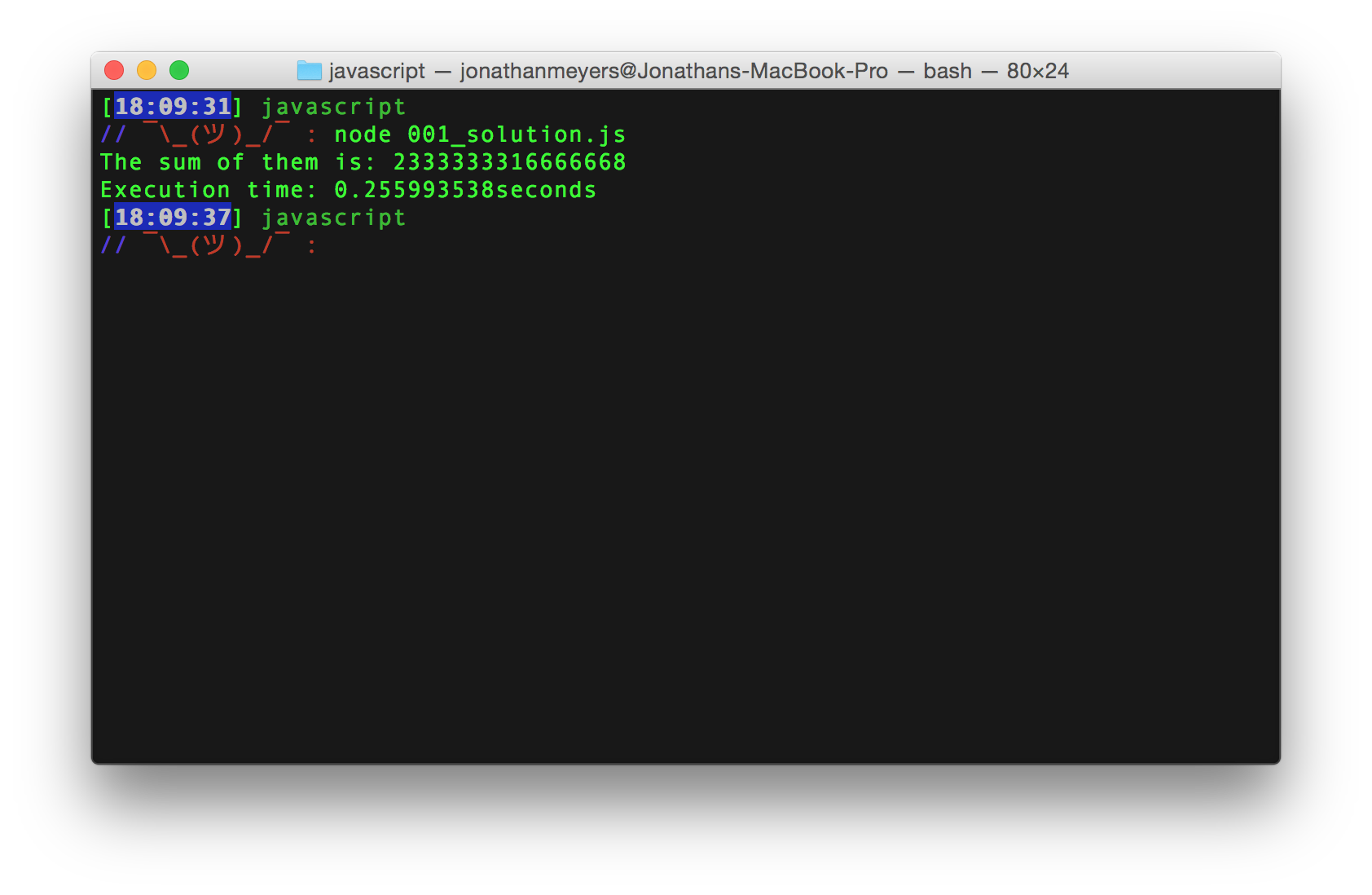
JAVASCRIPT WINS BY A LANDSLIDE!!!!
I was fascinated by the results of these two tests. Even though they confirmed that Javascript is very fast, the tests uncovered an interesting interpreter behavior. My first guess as to why Ruby performs better on small calculations is start up time. Node may have a slower initialization time, which would lead to slower performance on small calculations, but improved performance on large calculations where the full wrath of V8 can be unleashed. I look forward to testing more Project Euler problems and taking a look under the hood of both node and YARV in the near future.
Be sure to check out the github repo for this project and submit a pull request for your race.